#pandas rename coloumn #change column names pandas
There are several methods to rename column in Pandas or change column name of Pandas Dataframe in Python. In this article, you will see some of these methods being applied followed by some practical tips for using them.
Create a basic data frame and rename a column in pandas
# Creating a basic DataFrame
import pandas as pd
df = pd.DataFrame({
'name': ['Aryan', 'Rohan', 'Riya', 'Yash', 'Siddhant'],
'Tpye': [
'Full-time Employee', 'Intern', 'Part-time Employee',
'Part-time Employee', 'Full-time Employee'
],
'Dept':
['Administration', 'Technical', 'Management', 'Technical', 'Technical'],
'Salary': [20000, 5000, 10000, 10000, 20000]
})
df
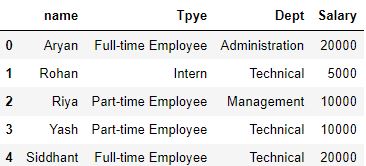
The column names of the above DataFrame are not descriptive or are inappropriate. Let’s see how to renames the columns.
pandas.DataFrame.rename
The pandas.DataFrame.rename
method is the main method to rename the columns in a pandas DataFrame.
Purpose: To change or customize labels of a Pandas DataFrame.
Parameters:
– mapper: Dictionary or function. It is used to apply a particular function to transform the names of the columns.
– axis: Int or string (default: ‘index‘). Used to determine whether to apply the changes either to indices or column names by specifying the integer value 0 (for indices) or 1(for columns) or the string value (‘index’ for indices or ‘columns’ for columns).
– index: Dictionary or function. Alternative to specifying the axis value for changing indices. This parameter need not be passed if the value of the axis parameter is specified.
– columns: Dictionary or function. Alternative to specifying the axis value for changing column names.This parameter need not be passed if the value of the axis parameter is specified.
– copy: Boolean (default: True). For copying the underlying data.
– inplace: Boolean(default:False). If this parameter is set to False then a new DataFrame having the required changes will be created.
– level: Int or string. In case of multi-level index, the column name will be changed only at the specified level.
– errors: string (default: ‘ignore‘). If this parameter is set to ‘raise’ then a KeyError will be raised if the name to be changed does not exist at the specified level. If set to false, such cases will be ignored.
Returns:
A pandas DataFrame with the labels changed at the specified axis.
Although there are several parameters available in the rename
method, only a few of them are required for changing column names.
Renaming a single column
# Using df.columns to view the column names of the DataFrame
print('Before:', df.columns)
#OUTPUT

Let’s rename.
df.rename(columns={'name': 'Name'}, inplace=True)
# Passing a dictionary where the original column name is the key and the new column name is passed as the value to the key.
print('After:', df.columns)
#OUTPUT

Rename the multiple columns
The rename
method can also be used to rename multiple columns in pandas.
The original column names are passed as the keys in the dictionary and the new column names are passed as the values to the corresponding keys in the dictionary.
df.rename(columns={
"name": "Name",
"Tpye": "Type",
"Dept": "Department"
},
inplace=True)
print('After:', df.columns)
pandas.DataFrame.columns
We can directly assign a new list or a tuple of column names to a DataFrame. This can be useful to rename multiple columns in pandas at once.
print('Before:', df.columns)

# Directly assign a list of new column names as the column names of the dataset.
df.columns = ['Name', 'Type', 'Department', 'Salary']
print('After:', df.columns)

pandas.DataFrame.set_axis
The pandas.DataFrame.set_axis
method is used to change the index or column names. This method can also be used to rename the multiple columns at once in pandas by passing a list or tuple of new column names and setting the value of the axis parameter to 1.
print('Before:', df.columns)

# Pass a list of column names and set axis=1
df.set_axis(['Name', 'Type', 'Department', 'Salary'], axis=1, inplace=True)
print('After:', df.columns)

Practical Tips
- The
rename
method can be useful to rename a single column or multiple columns at once. However, do not forget to set the inplace parameter to ‘True’, so that the changes you make are applied on the dataframe. - The
rename
method can also be used to apply custom transformations on column names by using user-defined functions.
For example:
print('original column names:', df.columns)


- It is recommended to use the
columns
or theset_axis
method only when most or all of the column names need to be changed because the entire list of column names will have to be passed each time this method is used and it may be cumbersome to do so.
Test Your Knowledge
Q1: How can we change the column names using the pandas.DataFrame.rename
method?
A: The old column names and the new column names are passed as corresponding key-value pairs in a dictionary or a custom user-defined function can also be used to change the column names.
Q2: True of False: When using the pandas.DataFrame.columns
method, the column names are changed by passing a list containing only the new column names to the method.
A: False, the list passed to the function should contain the names of all the features in the dataset.
Q3: What should be the value of the axis parameter when changing column names using the pandas.DataFrame.set_axis
method?
A: 1
Q4: Identify the error in the given code and write the correct code for changing column names using the set_axis
method:
df.set_axis = (('Name', 'Type', 'Department', 'Salary'), axis=0)
A: df.set_axis = (('Name', 'Type', 'Department', 'Salary'), axis=1)
This article was contributed by Shreyansh.