Pandas series can be converted to a list using tolist()
or type casting method.
There can be situations when you want to perform operations on a list instead of a pandas object. In such cases, you can store the DataFrame columns in a list and perform the required operations. After that, you can convert the list back into a DataFrame.
In this article, you will learn how to use these methods to convert a pandas Series to a list followed by a few practical tips for using them.
Creating a pandas Series
Let’s create a simple pandas series as an example.
import pandas as pd
# Create the data of the series as a dictionary
data_ser = {'Name': 'Sony',
'Country of Origin': 'Japan',
'Revenue': 25000000000}
# Create the series
ser = pd.Series(data_ser)
ser
Name Sony
Country of Origin Japan
Revenue 25000000000
dtype: object
There are multiple other methods also to create a series apart from above.
How to use the tolist() method to convert pandas series to list
To convert a pandas Series to a list, simply call the tolist()
method on the series which you wish to convert.
# Convert the series to a list
list_ser = ser.tolist()
print('Created list:', list_ser)
Created list: ['Sony', 'Japan', 25000000000]
Converting a DataFrame column to list
The columns of a pandas DataFrame are also pandas Series objects. You can convert the columns into a list using the tolist()
method.
Let’s create a simple DataFrame to perform this operation as shown below.
# Create the data of the DataFrame as a dictionary
data_df = {'Name': ['Sony', 'Tencent', 'Nintendo', 'Microsoft', 'Activision Blizzard'],
'Country of Origin': ['Japan', 'China', 'Japan', 'USA', 'USA'],
'Revenue': [25000000000, 13900000000, 12100000000, 11600000000, 8100000000]}
# Create the DataFrame
df = pd.DataFrame(data_df)
df
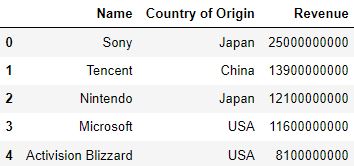
After loading/creating the DataFrame, use the tolist()
method on the selected column.
# Convert the name column of the DataFrame to a list
name_list = df['Name'].tolist()
print('Created list:', name_list)
print('Data type of the created list:', type(name_list))
Created list: ['Sony', 'Tencent', 'Nintendo', 'Microsoft', 'Activision Blizzard']
Data type of the created list: <class 'list'>
Using the type casting method to convert series to list
Typecasting is the process of converting the data type of an object to another data type.
You can directly perform type casting to convert a series to a list in pandas. All you have to do is pass the series to a list()
function
# Convert a pandas series to a list using type casting
list_ser = list(ser)
print('Created list:', list_ser)
print('Object type of the created list:', type(list_ser))
Created list: ['Sony', 'Japan', 25000000000]
Object type of the created list: <class 'list'>
You can also perform type casting to convert a DataFrame column to a list.
It is similar to using the tolist()
method wherein you select the column which is to be converted and then typecast it to a list.
# Convert a DataFrame column to a list using type casting
name_list = list(df['Name'])
print('Created list:', name_list)
print('Data type of the created list:', type(name_list))
Created list: ['Sony', 'Tencent', 'Nintendo', 'Microsoft', 'Activision Blizzard']
Data type of the created list: <class 'list'>
Practical Tips
- If you are converting a DataFrame column to a list, remember that you cannot convert it to a list and store it in the same variable. You need to store the list in a new variable.
- The
tolist()
method and the type casting methods work for pandas Series having nested objects as well. - The type casting method can work on any iterable whereas the
tolist()
method will be used only on those iterables on which it is explicitly called.
Test Your Knowledge
Q1: The tolist()
method can convert a DataFrame column to a list inplace. True or False?
Q2: You have a DataFrame df having columns col_1, col_2, and col_3. Write the code to convert col_2 to a list using the tolist()
method.
Answer: col_2_list = col_2.tolist()
Q3: Write the code to convert a pandas Series ser to a list using the type casting method.
Answer:Answer: list_ser = list(ser)
Q4: Write the code to convert a pandas Series ser to a list using the tolist()
method.
Answer: list_ser = ser.tolist()
>
This article was contributed by Shreyansh B and Shri Varsheni